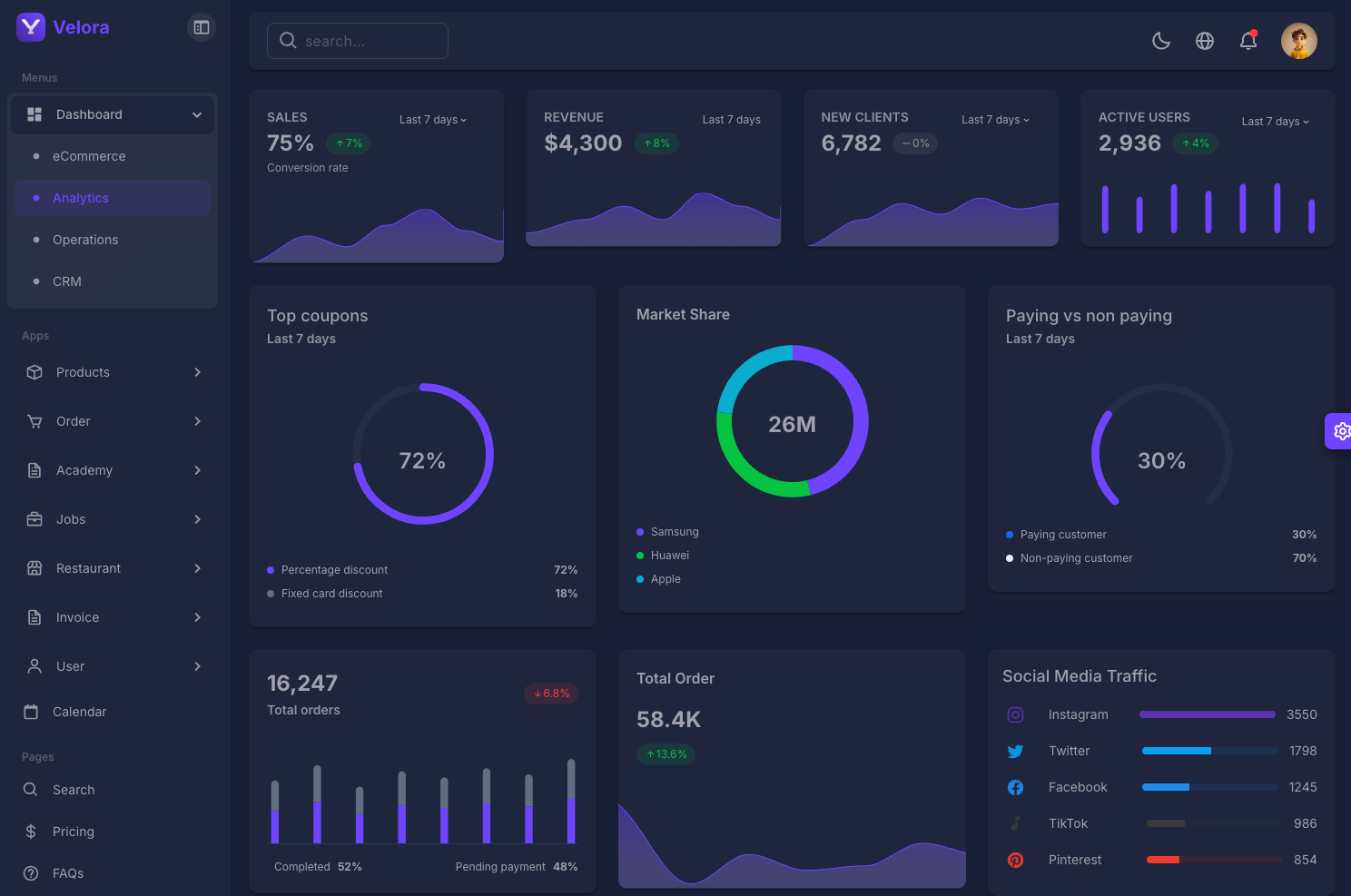
Laravel and Vue.js are a match made in developer heaven. Laravel, with its expressive syntax and powerful tools, makes backend development a breeze. Vue.js, on the other hand, shines when building interactive, dynamic user interfaces. Together, they create seamless applications. In this blog, we’ll walk through the process of building a scalable API-only backend with Laravel to supercharge your Vue.js applications. Ready? Let’s dive in!
Why Choose Laravel for Your API-Only Backend?
A Quick Intro to Laravel and Vue.js
Laravel is a popular PHP framework known for its elegant syntax and developer-friendly features. It’s great for building robust APIs. Vue.js, a progressive JavaScript framework, is perfect for creating user interfaces and SPAs (Single Page Applications).
Use Cases for API-Only Backends
Why go API-only? This approach is ideal for:
-
SPAs: Vue.js can focus entirely on the UI while Laravel handles the backend.
-
Mobile Apps: Share a single backend for both web and mobile clients.
-
Microservices: Modularize your system with separate, scalable components.
Why Laravel?
Laravel’s built-in tools—such as Eloquent ORM, authentication, and middleware—make it an excellent choice for API development. Plus, it’s backed by an active community and solid documentation.
Setting Up Laravel as an API-Only Backend
Step 1: Install Laravel
Start by installing Laravel using Composer:
composer create-project --prefer-dist laravel/laravel api-backend
Navigate to your project directory:
cd api-backend
Step 2: Configure Routes
Laravel provides an api.php file specifically for API routes. Add your routes here:
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
Step 3: Middleware for APIs
Enable middleware for features like:
-
Authentication: Use Sanctum or Passport.
-
Rate Limiting: Prevent abuse by limiting requests.
Update the app/Http/Kernel.php file if needed.
Designing a RESTful API with Laravel
Best Practices for API Route Design
- Use resourceful routing for cleaner code:
-
Route::apiResource('posts', PostController::class);
-
Group similar routes and use versioning:
-
Route::prefix('v1')->group(function () { Route::apiResource('posts', PostController::class); });
Use Controllers and Resource Classes
Create a controller for handling your logic:
php artisan make:controller PostController --api
Use resource classes for consistent responses:
php artisan make:resource PostResource
Example:
return new PostResource($post);
Securing the API
Token-Based Authentication
Laravel Sanctum is perfect for API authentication. Install it:
composer require laravel/sanctum
Publish the config:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Add middleware to api.php routes:
Route::middleware('auth:sanctum')->group(function () {
Route::get('/user', [UserController::class, 'index']);
});
CORS Setup
To allow your Vue.js app to interact with the API, configure CORS in the config/cors.php file. Set supports_credentials to true if needed.
Role-Based Middleware
Define middleware to handle user roles and permissions:
php artisan make:middleware RoleMiddleware
Integrating the Laravel API with Vue.js
Setting Up Axios or Fetch API
In your Vue.js project, install Axios:
npm install axios
Set up Axios globally in main.js:
import axios from 'axios';
axios.defaults.baseURL = 'http://localhost/api';
Handling Authentication
Store tokens securely in localStorage or cookies. Example:
axios.post('/login', credentials).then(response => {
localStorage.setItem('token', response.data.token);
});
Managing State with Vuex or Pinia
Use Vuex or Pinia to manage API state efficiently:
const store = createStore({
state: {
user: null
},
mutations: {
setUser(state, user) {
state.user = user;
}
},
actions: {
fetchUser({ commit }) {
axios.get('/user').then(response => {
commit('setUser', response.data);
});
}
}
});
Error Handling and Validation
Request Validation in Laravel
Validate API inputs with request classes:
php artisan make:request StorePostRequest
Example:
public function rules()
{
return [
'title' => 'required|string|max:255',
'body' => 'required|string',
];
}
Structured Error Responses
Return consistent error formats:
return response()->json(['error' => 'Invalid request'], 400);
Handle Errors Gracefully in Vue.js
Show user-friendly messages for API errors:
axios.get('/data').catch(error => {
console.error(error.response.data.message);
});
Optimizing the API for Performance
Query Scopes and Eager Loading
Avoid N+1 queries with eager loading:
$posts = Post::with('comments')->get();
Cache API Responses
Use Laravel Cache for frequently accessed data:
Cache::remember('posts', 60, function () {
return Post::all();
});
Pagination for Large Datasets
Paginate responses to improve performance:
return Post::paginate(10);
Deploying the Laravel API
Preparing for Production
Set APP_ENV to production and enable debugging restrictions. Use HTTPS for secure communication.
Hosting Options
Laravel Forge or VPS providers like Linode and DigitalOcean work great for hosting.
Deploying Frontend and Backend
Serve the Vue.js app and API on subdomains or separate domains for scalability.
Wrapping Up
Building a scalable API-only backend with Laravel for Vue.js is not just efficient but also rewarding. By separating the frontend and backend, you get flexibility, better performance, and a modular codebase. Once you’ve set up your API, start exploring ways to scale, monitor, and enhance security. The sky’s the limit!