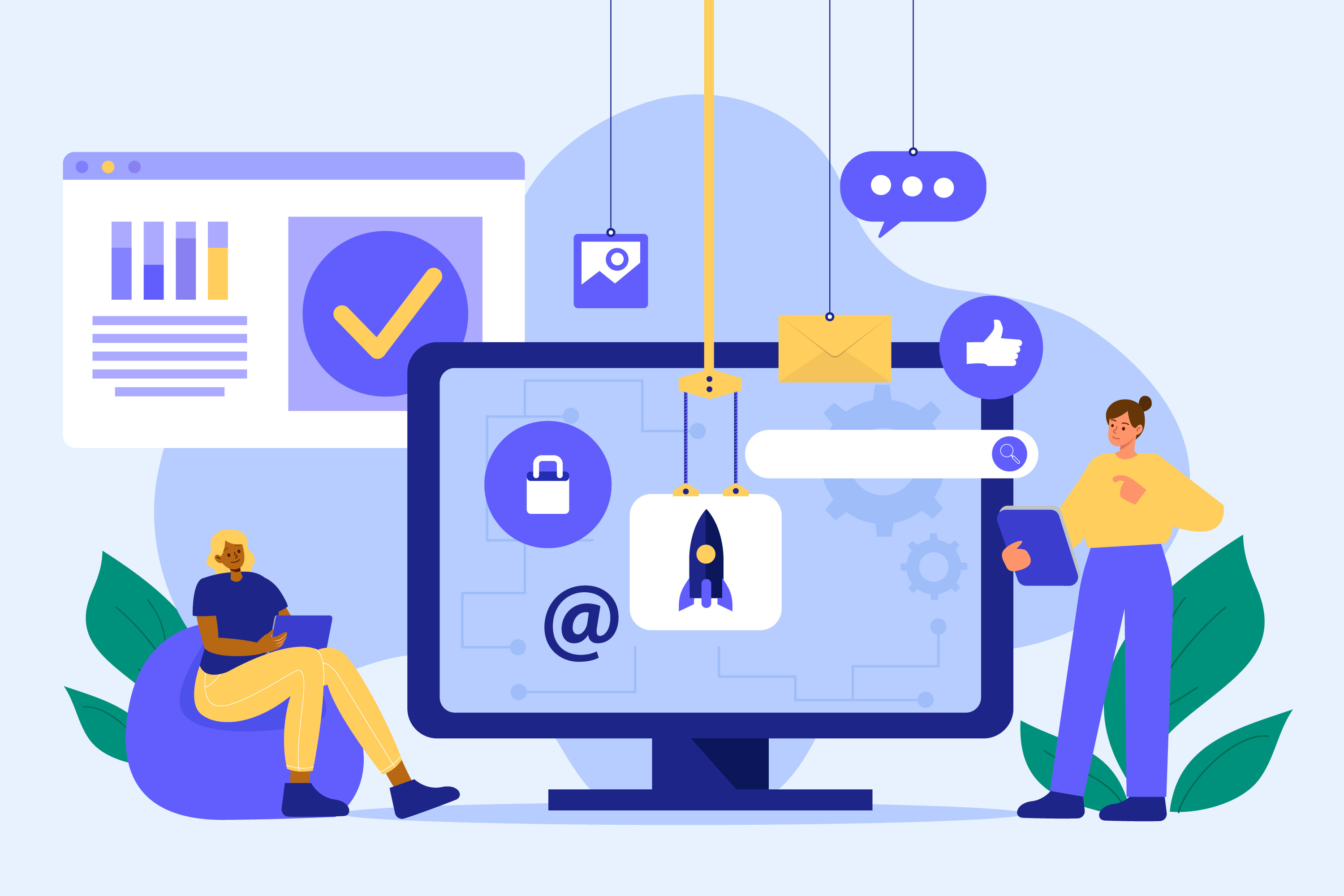
When it comes to building web applications, Laravel is one of the most popular PHP frameworks out there. But with great power comes great responsibility—securing your Laravel application is crucial. If you’re a beginner, don’t worry. This guide will walk you through some of the best practices to keep your Laravel app safe from common security threats. Let’s dive in!
1. Keep Your Laravel Version Updated
Why Updates Matter
Laravel releases frequent updates to patch vulnerabilities and improve security. Running an outdated version of Laravel is like leaving your front door unlocked—you’re inviting trouble!
How to Stay Updated
Regularly check the Laravel website or GitHub repository for updates. Use Composer to upgrade your framework:
composer update
Don’t forget to test your application after updates to ensure nothing breaks.
2. Leverage Built-In Authentication
Laravel Makes Authentication Easy
Authentication is your app’s first line of defense. Luckily, Laravel’s built-in authentication system is a breeze to set up. Whether you’re using email/password, social logins, or two-factor authentication, Laravel has you covered.
Steps to Implement
Run the following Artisan command to scaffold authentication:
php artisan make:auth
Or, if you're using Laravel Breeze or Jetstream, follow their documentation for a more modern setup. Always encourage users to create strong passwords and enable multi-factor authentication (MFA).
3. Use Environment Variables Wisely
Protect Sensitive Information
Your .env file holds sensitive data like database credentials, API keys, and mail server settings. Exposing this file is a big no-no.
Best Practices
-
Never commit .env to your Git repository.
-
Use tools like GitGuardian to scan for exposed secrets.
-
For production, store sensitive data in a secure location like AWS Secrets Manager or HashiCorp Vault.
4. Secure Your Routes
Public vs. Private Routes
Not all routes in your application should be public. Protect sensitive routes with middleware.
Middleware in Action
Laravel provides middleware like auth and verified to control access. For example:
Route::get('/dashboard', [DashboardController::class, 'index'])->middleware('auth');
You can even create custom middleware for more specific use cases, like role-based access control.
5. Prevent SQL Injection
Use Eloquent and Query Builder
Laravel’s Eloquent ORM and query builder automatically use parameter binding, which protects against SQL injectio
Avoid Raw Queries
While raw SQL queries can be powerful, they’re also risky. If you must use them, always bind your parameters:
DB::select('SELECT * FROM users WHERE id = ?', [$id]);
But honestly, stick with Eloquent when you can. It’s safer and easier to read.
6. Enable CSRF Protection
What is CSRF?
Cross-Site Request Forgery (CSRF) tricks users into performing actions they didn’t intend. Laravel has built-in CSRF protection to guard against this.
How Laravel Handles CSRF
Every form in Laravel includes a hidden CSRF token:
<form method="POST" action="/submit">
@csrf
<!-- Your form fields -->
</form>
Never disable CSRF protection unless you absolutely know what you’re doing.
7. Sanitize User Input
Validate Everything
User input can be a gateway for attacks like XSS (Cross-Site Scripting). Laravel’s validation rules help sanitize and validate inputs effortlessly.
Example Validation
$request->validate([
'email' => 'required|email',
'name' => 'required|string|max:255',
]);
Always escape output using the {{ }} blade directive to prevent XSS.
8. Use HTTPS Everywhere
Encrypt Data in Transit
Using HTTPS encrypts the data exchanged between your users and your application. It’s an absolute must for any modern web application.
How to Enable HTTPS
-
Get an SSL certificate (many hosting providers offer this for free).
-
Update your .env file to force HTTPS:
-
APP_URL=https://yourdomain.com
- Use middleware to redirect HTTP traffic to HTTPS:
-
\App\Http\Middleware\RedirectToHttps::class
9. Monitor and Log Activity
Stay Alert
Monitoring helps you detect suspicious activity before it becomes a full-blown issue. Laravel’s logging system makes this straightforward.
How to Set Up Logging
Configure logging in your config/logging.php file. Use services like Laravel Telescope or third-party tools like Sentry for advanced monitoring.
10. Backup Regularly
Why Backups Are Essential
Even with the best security measures, things can go wrong. Regular backups ensure you can recover quickly.
Tools for Backups
Laravel has packages like Spatie’s Laravel Backup to automate backups:
composer require spatie/laravel-backup
Set it up to back up your database and files to a secure location.
Wrapping Up
Securing your Laravel application isn’t optional; it’s a responsibility. By following these best practices, you’ll create a robust and secure application that stands up to common threats. Remember, security is an ongoing process, so stay updated and vigilant.
Happy Coding!