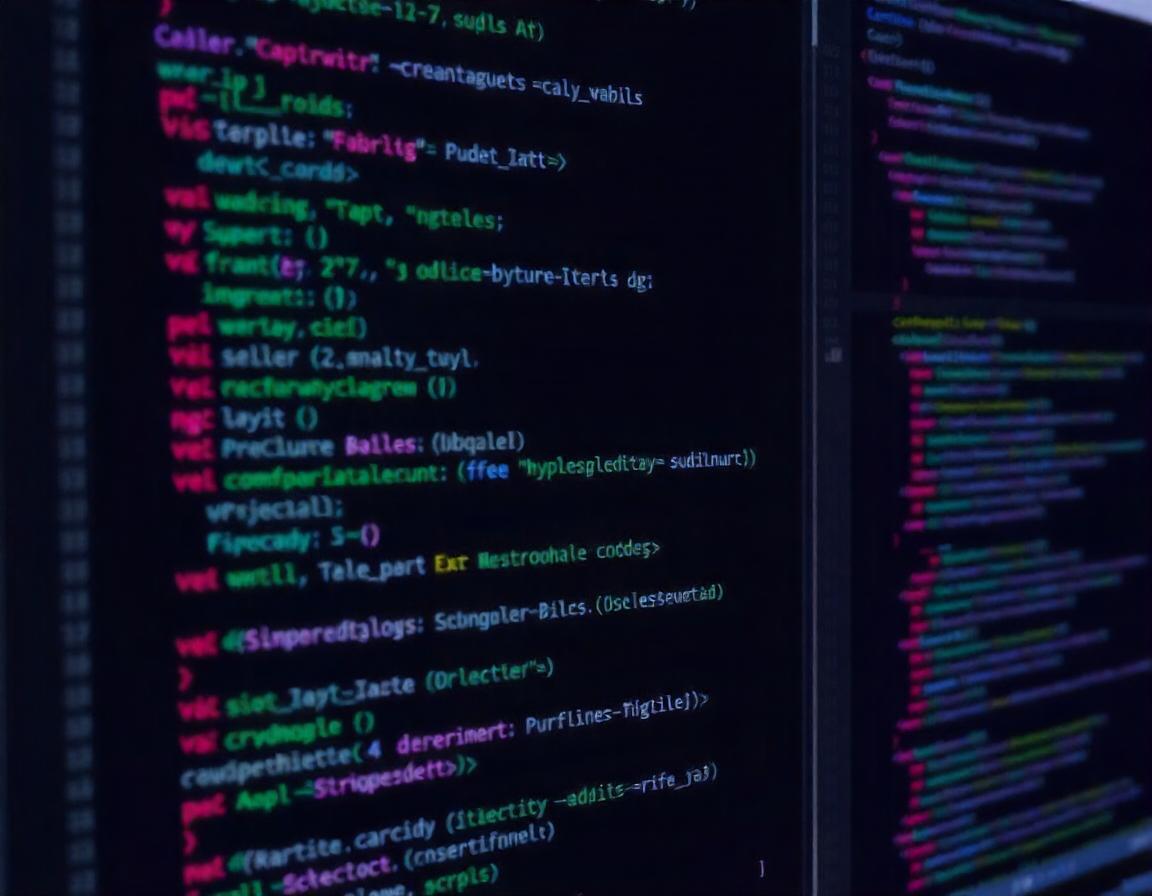
Authentication is one of the pillars of modern web development. Whether it’s allowing users to log in or restricting access to specific parts of an application, a reliable authentication system ensures both security and a seamless user experience.
Laravel, known for its developer-friendly approach, takes the complexity out of authentication. It offers tools and packages that help developers set up secure and scalable systems without reinventing the wheel. If you’re a developer looking to master Laravel’s authentication features, this blog is for you. We'll break it down step-by-step, covering both the basics and advanced options.
Introduction
Imagine logging into a web app, confident that your data is safe. That’s the essence of a great authentication system—security and simplicity. For developers, however, building such systems from scratch can be a daunting task. Enter Laravel.
Laravel's motto, "love beautiful code," extends to its authentication features. It doesn’t just make it easy; it makes it enjoyable. Whether you're working on a small side project or a full-scale enterprise solution, Laravel has you covered with its built-in tools and customizable options.
Exploring Authentication Options in Laravel
Laravel provides several ways to implement authentication, each tailored to different levels of complexity. Let’s start with the basics and then explore how Laravel’s packages—Breeze and Jetstream—help you scale up as your project grows.
Default Laravel Authentication
Laravel’s default authentication system comes ready to use with features like login, registration, password reset, and email verification. It’s easy to get started. With just a few commands, Laravel generates everything you need, including database migrations, controllers, and views.
Why developers love it:
- Time-saving: No need to code basic authentication features from scratch.
- Customizable: You can tweak the generated files to match your app’s design and logic.
Authentication Packages for Enhanced Features
Laravel offers two main packages for authentication scaffolding:
- Laravel Breeze: A simple, no-frills solution for quick implementation.
- Laravel Jetstream: A more advanced option for modern applications requiring features like two-factor authentication and API tokens.
Detailed Comparison of Packages
Let’s compare Laravel Breeze and Jetstream so you can decide which fits your project best.
Laravel Breeze: Quick and Minimal
Think of Laravel Breeze as your "starter pack" for authentication. It’s perfect for projects where you want a lightweight, functional authentication system without unnecessary complexity.
What makes it great:
- Comes with basic features: login, registration, password reset, and email verification.
- Uses Tailwind CSS for styling, so it’s modern and easy to extend.
- Super simple to set up:
composer require laravel/breeze --dev
php artisan breeze:install
npm install && npm run dev
php artisan migrate
When to use Breeze:
- You’re working on a smaller app with straightforward authentication needs.
- You want to quickly prototype an idea without spending time on complex setups.
Laravel Jetstream: Advanced and Feature-Rich
Jetstream builds on Breeze but takes authentication to the next level. If you’re building a more complex application, this is the tool you need.
Key Features:
- Two-factor authentication: Adds an extra layer of security for your users.
- API token management: Works seamlessly with Laravel Sanctum for API-based apps.
- Team management: Great for multi-tenant applications.
- Offers options for Livewire (Blade components) or Inertia.js (Vue.js) front-ends.
Installation:
composer require laravel/jetstream
php artisan jetstream:install livewire # Or use inertia
npm install && npm run dev
php artisan migrate
When to use Jetstream:
- You’re building an app that requires advanced user management (e.g., teams or roles).
- Your app has a modern front-end powered by Vue.js or you prefer Livewire.
Comparison Table
Feature | Laravel Breeze | Laravel Jetstream |
Complexity | Simple and minimal | Advanced and comprehensive |
Front-end Framework | Tailwind CSS | Tailwind with Livewire/Inertia |
Two-Factor Auth | No | Yes |
API Tokens | No | Yes (via Sanctum) |
Team Management | No | Yes |
Additional Authentication Features
Laravel isn’t just about scaffolding. Its additional features give you the tools to build secure, flexible authentication systems:
1. Role-Based Access Control (RBAC)
With role-based access control, you can define what users with different roles (like admin, editor, or viewer) are allowed to do. Using a popular package like Spatie Laravel Permission, implementing RBAC becomes a breeze.
2. Middleware for Authentication and Authorization
Laravel’s middleware makes route protection easy. For instance:
Route::get('/dashboard', [DashboardController::class, 'index'])->middleware('auth');
Want to allow only admins? Use the can
middleware:
Route::get('/admin', [AdminController::class, 'index'])->middleware('can:manage-users');
3. Token-Based Authentication
For API-based projects, Laravel offers:
- Sanctum: Perfect for lightweight API token management.
- Passport: A more robust option implementing OAuth2 for complex use cases.
Implementation Tips
Creating an authentication system isn’t just about functionality—it’s about security and user experience. Here are a few tips to keep in mind:
1. Protect Sensitive Data
- Always hash passwords using Laravel’s built-in tools:
use Illuminate\Support\Facades\Hash;
$user->password = Hash::make($request->password);
- Use HTTPS to encrypt data in transit.
2. Validate User Input
Validation is your first line of defense against malicious data:
$request->validate([
'email' => 'required|email',
'password' => 'required|min:8',
]);
3. Stay Updated
Laravel’s ecosystem evolves quickly. Keep your dependencies up-to-date to benefit from the latest security patches and features.
Conclusion
Laravel’s authentication tools are as versatile as they are powerful. Whether you’re just starting or scaling up, Laravel Breeze and Jetstream offer tailored solutions for your needs. Combined with middleware, role-based access, and API authentication, Laravel makes it easy to build secure systems while focusing on your app’s core functionality.
Choosing the Right Tool
- Use Laravel Breeze for simple, lightweight projects.
- Go with Laravel Jetstream if your app demands advanced features like two-factor authentication or team management.